ChatGPT: An Impressive Language Model! [14+ Examples]
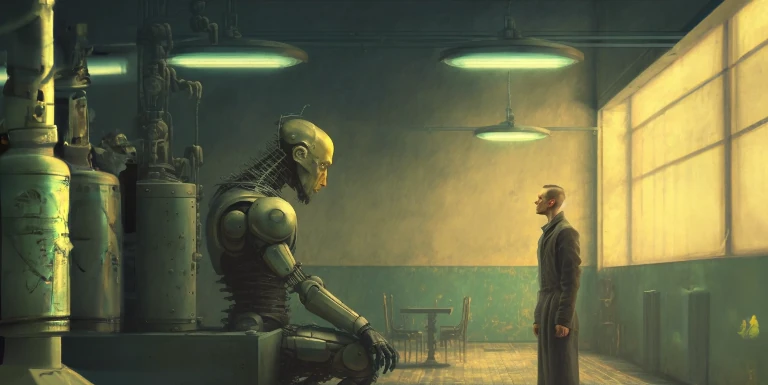
If you’ve been anywhere near Twitter or tech news for the past two weeks, you’ve seen talk about ChatGPT all over the place. In case you haven’t heard already, ChatGPT is the latest AI large language model from OpenAI, released November 30th, 2022.
In this post I’ll show you (impressive) examples of how you can use it and ways it can be useful for you. I’ll also share some tips and tricks I’ve learned by tinkering around with it as well as a list of resources if you wish to learn more.
Introduction⌗
ChatGPT is known as what’s called Language Models, which are AI models specifically designed for Natural Language Processing applications such as:
- Machine translation
- Text comprehension
- Information retrieval
- Summarization
- Classification
- Text generation
- Answering questions
Among other applications.
Timeline⌗
Language models have come a long way in the few past years, in fact the rate of progress in AI research is so fast, you can barely even keep up. So To contextualize ChatGPT and for a better idea of how we got here, here’s a (non-exhaustive) timeline of notable events relating to language models:
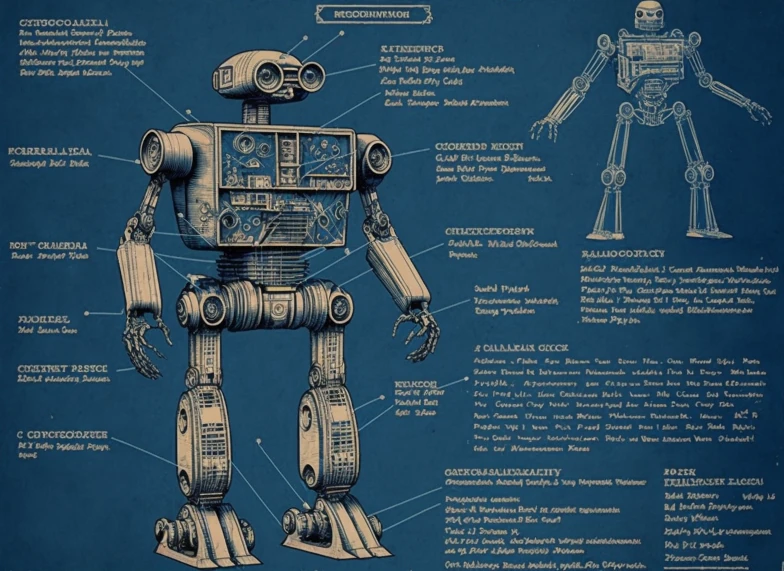
2017⌗
June
— A research team at Google publishes a paper introducing Transformer, a novel neural network architecture.
2018⌗
June
— OpenAI introduces GPT (paper).November
— Google open-sources BERT (paper), a pre-trained Natural Language Processing model.
2019⌗
February
— OpenAI publishes GPT-2 (paper), an improvement on GPT capable of generating coherent paragraphs of text, it also achieves better performance on text comprehension tasks.October
— Google starts using BERT to improve the accuracy of search results.
2020⌗
May
— OpenAI publishes a paper introducing GPT-3, a much larger model than its predecessor GPT-2.June
— OpenAI launches an API allowing third-parties to build applications on top of OpenAI’s models such as GPT-3.September
— OpenAI publishes a paper detailing their approach of using RLHF to achieve better results for generating text summaries.
2021⌗
June
— OpenAI publishes a paper showcasing their results for improving language model behavior by training on a curated dataset. They’ve fine-tuned GPT-3 on a 120KB dataset which is about 0.000000211% of the original training data.August
— OpenAI launches Codex (paper), an AI system that translates natural language to code. It’s the same model that powers Github Copilot.September
— OpenAI publishes a paper showcasing their technique of fine-tuning a GPT-3 model to summarize entire books. You can play with the demo they published for this model for a better idea of how it performs.December
— OpenAI adds the possibility to fine-tune a GPT-3 model via their API using your own data. The fine-tuned model is deployed on their API and you can use it to improve results and lower costs.December
— OpenAI demonstrates WebGPT (paper), a fine-tuned GPT-3 model with web access (via Bing’s Web Search API). The goal is to answer open-ended questions using a text-based web browser while mimicing how a human researches questions online. It also cites the sources for the answers it makes.
2022⌗
January
— OpenAI introduces InstructGPT (paper), a fine-tuned GPT-3 model (using RLHF) to better follow instructions and achieve better alignment with users’ intents.March
— OpenAI adds Editing & Insertion capabilities to GPT-3.November
— OpenAI introduces ChatGPT, a model trained to interact in a conversational way which works great as a chatbot. It has the capability to answer questions (and followup questions) while taking the context into consideration, it also admits its mistakes, challenges incorrect statements and refuses inappropriate requests. OpenAI opened up access to try ChatGPT as a “Free Research Preview”.
Learn More⌗
Videos⌗
But How Does ChatGPT Actually Work?: Explainer video from Till Musshoff
Large Language Models and the Future of AI: Excellent talk by Connor Leahy from EleutherAI. It provides a good overview of large language models and their potential.
Articles⌗
- A Brief Timeline of NLP from Bag of Words to the Transformer Family: A more detailed timeline of NLP developments.
- BERT Explained: State of the art language model for NLP.
- OpenAI GPT-3: Everything You Need to Know: This provides a good overview of how GPT-3 is different and what are some of its possible applications.
- The GPT-3 Architecture, on a Napkin: GPT-3 architecture explained with lots of diagrams.
Discussions⌗
Examples⌗
Now that we’ve got a good understanding of what ChatGPT is and a timeline that shows how we got here, let’s have some fun playing around with ✨ ChatGPT ✨!
Before we proceed, please note I’ll be copying the output I get from ChatGPT as text here for better readability and accessiblity (instead of screenshotting everything), I’ll also share a link for each example on ShareGPT. Here’s the convention used for the following examples:
🤔 > The prompt I write, fully human-made text.
🤖 > ChatGPT's output, machine-produced.
I might also use [...]
to truncate ChatGPT’s output if it’s not necessary to
clarify a point. Output tends to get long.
Other than that, I will copy ChatGPT’s output as is, unmodified.
With that out of the way, let’s see the examples!
Programming and Code-Related Uses⌗
ChatGPT has shown impressive capabilities when it comes to generating code, explaining code, writing documentation and providing help debugging.
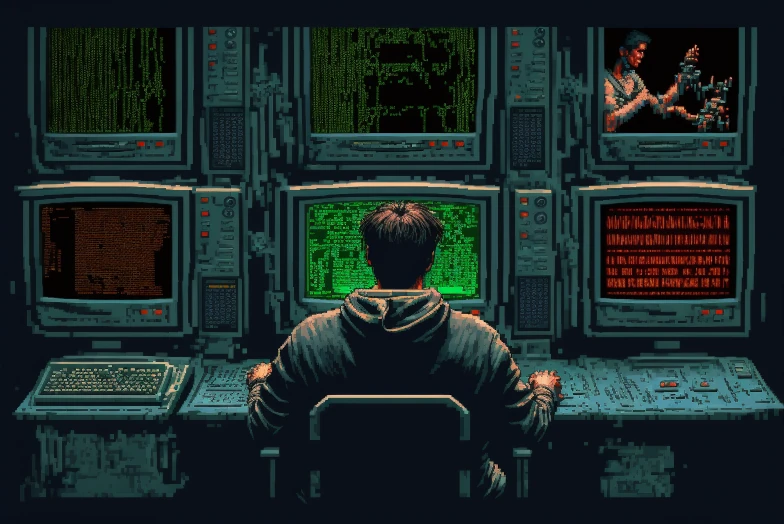
Jump to:
Example 1: Generate a Bash script⌗
🤔 > Make a bash script that takes a program name as an argument and checks if there's a running process for it using pgrep
🤖 > To create a bash script that takes a program name as an argument and checks if there's a running process for it using pgrep, you can use the following code:
#!/bin/bash
# Check if the user provided a program name as an argument
if [ $# -eq 0 ]; then
# If not, print an error message and exit
echo "Error: Please provide a program name as an argument."
exit 1
fi
# Use pgrep to search for a running process with the given name
pgrep $1
# Check the exit code of the previous command
if [ $? -eq 0 ]; then
# If the exit code is 0, that means a process was found, so print a message
# indicating that the program is running
echo "The program '$1' is currently running."
else
# If the exit code is not 0, that means no process was found, so print a
# message indicating that the program is not running
echo "The program '$1' is not currently running."
fi
🤖 > Save the code to a file named `check_program.sh`, make the file executable by running `chmod +x check_program.sh`, and then you can run the script by calling `./check_program.sh` followed by the name of the program you want to check. For example:
./check_program.sh bash
🤖 > This will search for a running process with the name "bash" and print a message indicating whether or not it is running.
Okay that’s a great start, however when you run this, if the program is running
it also outputs the process IDs for the program. I also want the script to exit
with a non-zero status code, let’s see if we can get it to update the script to
match our needs.
🤔 > Thanks, can you also not show the process IDs if the program is running? And if the program is not running, exit with a non-zero status code
🤖 > To modify the script to not show the process IDs when the program is running and to exit with a non-zero status code when the program is not running, you can use the following code:
#!/bin/bash
# Check if the user provided a program name as an argument
if [ $# -eq 0 ]; then
# If not, print an error message and exit
echo "Error: Please provide a program name as an argument."
exit 1
fi
# Use pgrep to search for a running process with the given name
pgrep $1 &> /dev/null
# Check the exit code of the previous command
if [ $? -eq 0 ]; then
# If the exit code is 0, that means a process was found, so print a message
# indicating that the program is running
echo "The program '$1' is currently running."
else
# If the exit code is not 0, that means no process was found, so print a
# message indicating that the program is not running and exit with a non-zero
# status code
echo "The program '$1' is not currently running."
exit 1
fi
🤖 > This updated script will not show the process IDs when the program is running, and it will exit with a non-zero status code when the program is not running. Save the code to a file named `check_program.sh`, make the file executable by running `chmod +x check_program.sh`, and then you can run the script as before by calling `./check_program.sh` followed by the name of the program you want to check. For example:
./check_program.sh bash
🤖 > This will search for a running process with the name "bash" and print a message indicating whether or not it is running. If the program is not running, the script will exit with a non-zero status code.
A little repetitive, but that sure gave us what we asked for!
As you can see, with minimal effort you can quickly generate working scripts,
or at least get a very good starting point. I can see this being super helpful
to avoid spending too much time on boilerplate code.
Example 2: Explain code and fix possible errors⌗
See it on ShareGPTI wanted to test its capabilities in explaining code and helping out with identifying possible issues and adding error handling. So I first asked ChatGPT to write a python script:
🤔 > Write a python script that fetches a popular quote form an API endpoint, it then appends the quote to a file
🤖 > Here is a python script that fetches a popular quote from an API endpoint and appends it to a file:
import requests
# API endpoint for fetching popular quotes
QUOTE_API_ENDPOINT = 'https://api.quotable.io/random'
# file to append the quote to
QUOTE_FILE = 'quotes.txt'
# fetch a quote from the API endpoint
response = requests.get(QUOTE_API_ENDPOINT)
# parse the response as JSON
quote = response.json()
# extract the quote text from the JSON object
quote_text = quote['content']
# append the quote to the file
with open(QUOTE_FILE, 'a') as f:
f.write(quote_text)
f.write('\n')
print(f'Successfully appended quote to {QUOTE_FILE}')
🤖 > This script uses the requests library to make an HTTP GET request to the API endpoint, which returns a JSON object containing a quote. The script then parses the JSON response and extracts the quote text, and appends it to a file. Note that this script is just an example and may not work as-is, since it is not connected to a real API endpoint. You will need to provide your own API endpoint and modify the script accordingly.
I then copied the Python code, removed all the comments, started a new session,
pasted the code and asked ChatGPT to explain the code and add comments.
See it on ShareGPT
🤔 > I don't understand what the following python script does, can you add comments in the code and also explain what it's doing?
import requests
QUOTE_API_ENDPOINT = 'https://api.quotable.io/random'
QUOTE_FILE = 'quotes.txt'
response = requests.get(QUOTE_API_ENDPOINT)
quote = response.json()
quote_text = quote['content']
with open(QUOTE_FILE, 'a') as f:
f.write(quote_text)
f.write('\n')
print(f'Successfully appended quote to {QUOTE_FILE}')
🤖 > This script uses the `requests` library to make a GET request to the `QUOTE_API_ENDPOINT` which is a URL for a quote API. This API returns a quote in JSON format, which the script then parses to extract the quote text. The quote text is then written to the `QUOTE_FILE`, which is specified as `'quotes.txt'`, using the `with open` statement in Python. This will open the file in append mode, meaning that the quote will be appended to the end of the file. Finally, the script prints a message indicating that the quote was successfully appended to the file. Here is the script with added comments:
import requests # Import the requests library
QUOTE_API_ENDPOINT = 'https://api.quotable.io/random' # URL for the quote API
QUOTE_FILE = 'quotes.txt' # File to store the quotes
# Make a GET request to the quote API
response = requests.get(QUOTE_API_ENDPOINT)
# Parse the JSON response to extract the quote text
quote = response.json()
quote_text = quote['content']
# Append the quote text to the quotes file
with open(QUOTE_FILE, 'a') as f:
f.write(quote_text)
f.write('\n')
# Print a success message
print(f'Successfully appended quote to {QUOTE_FILE}')
Yep, it perfectly explains what the script is doing.
Now let’s try and see if
it can help us identify possible issues with the code and maybe even implement
a fix for us?
🤔 > Can you find any possible bugs in this code ?
🤖 > There are no obvious bugs in this code, but there are a few potential issues that could arise. For example, if the `QUOTE_API_ENDPOINT` is not available or is not responding, the `requests.get()` call will raise an exception, which will cause the script to crash. Additionally, if the JSON response from the API does not contain a `'content'` field, trying to access `quote['content']` will raise a `KeyError`. Here is a modified version of the script that includes error handling for these cases:
import requests # Import the requests library
QUOTE_API_ENDPOINT = 'https://api.quotable.io/random' # URL for the quote API
QUOTE_FILE = 'quotes.txt' # File to store the quotes
try:
# Make a GET request to the quote API
response = requests.get(QUOTE_API_ENDPOINT)
# Parse the JSON response to extract the quote text
quote = response.json()
quote_text = quote['content']
except requests.exceptions.RequestException as e:
# Handle any errors that occurred while making the request
print(f'An error occurred while making the request: {e}')
except KeyError as e:
# Handle cases where the JSON response does not have the expected format
print(f'Invalid response from the API: {e}')
else:
# Append the quote text to the quotes file
with open(QUOTE_FILE, 'a') as f:
f.write(quote_text)
f.write('\n')
# Print a success message
print(f'Successfully appended quote to {QUOTE_FILE}')
So it correctly identified troublesome code, implemented safeguards and added descriptive error messages. Isn’t that something!
Are you enjoying this post?
Get notified when I publish new posts:
Writing / Marketing⌗
ChatGPT is capable of producing great results when it comes to writing / text generation related tasks!
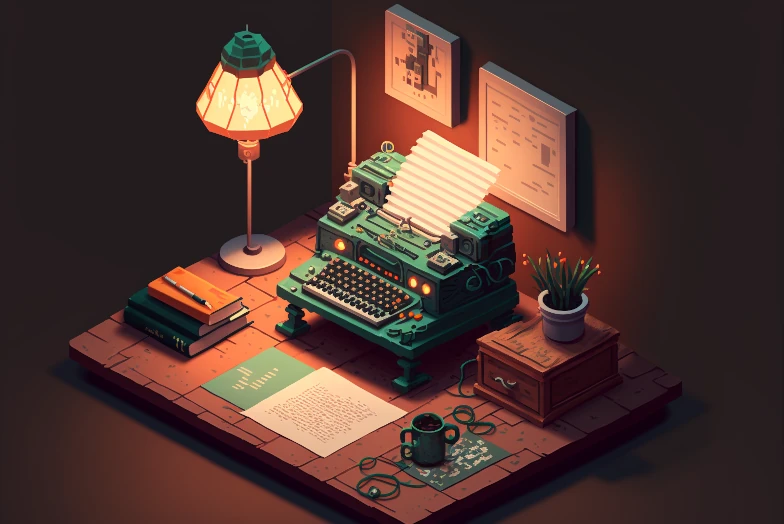
Jump to:
Example 1: Generate a draft for a blog post⌗
🤔 > Generate a draft for a blog post about AI large language models and their use-cases, divide the draft into sections with headers and subheaders
🤖 > Blog Post Draft: Title: The Power of AI Large Language Models: Exploring their Use-Cases Intro: AI large language models, such as GPT-3 and BERT, have taken the world of natural language processing (NLP) by storm. With the ability to understand and generate human-like language, these models have the potential to revolutionize a variety of industries and applications. In this blog post, we will explore some of the most promising use-cases for AI large language models and discuss how they can benefit businesses and individuals alike. Section 1: Text Generation One of the most exciting applications of AI large language models is their ability to generate human-like text. This can be used in a variety of ways, such as automatically generating content for websites or social media, or creating personalized emails and responses. By using these models, businesses can save time and resources on content creation, while still providing high-quality, engaging material. Subsection 1: Content Creation One of the most common uses for text generation is the creation of content for websites and social media. With AI large language models, businesses can automatically generate articles, blog posts, and other written material, saving time and resources on content creation. Additionally, these models can be trained on a specific brand's tone and style, ensuring that the generated content is consistent with the company's voice. Subsection 2: Personalized Communications Another promising use for text generation is the creation of personalized emails and responses. AI large language models can be trained to understand a person's individual preferences and needs, and then generate custom-tailored responses that are engaging and relevant. This can be especially useful for customer service, where the ability to quickly and accurately respond to individual inquiries can improve the customer experience and build brand loyalty. Section 2: Sentiment Analysis In addition to generating text, AI large language models can also be used to analyze and understand human emotions and opinions. This is known as sentiment analysis, and it can be incredibly useful for a variety of applications. For example, businesses can use sentiment analysis to understand how their customers feel about their products or services, and then use that information to improve their offerings and increase customer satisfaction. Subsection 1: Customer Feedback One of the most common uses for sentiment analysis is the analysis of customer feedback. By using AI large language models to analyze customer reviews and comments, businesses can gain a better understanding of what their customers like and dislike about their products or services. This can help them identify areas for improvement and make changes that will increase customer satisfaction. Subsection 2: Social Media Monitoring Another potential use for sentiment analysis is the monitoring of social media. By using AI large language models to analyze social media posts and comments, businesses can gain insight into how their brand is perceived by the public. This can help them identify potential issues or concerns, and take steps to address them before they become major problems. Conclusion: AI large language models have the potential to revolutionize a variety of industries and applications. Whether it's generating high-quality content, providing personalized communications, or analyzing sentiment, these models have the power to benefit businesses and individuals alike. With their ability to understand and generate human-like language, AI large language models are poised to become an essential tool for anyone looking to improve their NLP capabilities.
Example 2: Generate marketing copy⌗
🤔 > Write a short marketing copy for an app that generates profile pictures using AI in any style you want
🤖 > Are you tired of using the same old profile picture on your social media accounts? With our new app, you can generate unique and personalized profile pictures using AI technology. Choose from a variety of styles to suit your mood or personality, and let our advanced algorithms create a one-of-a-kind image for you. Say goodbye to boring profile pictures and stand out from the crowd with AI-generated images from our app. Try it now!
🤔 > Give me 4 bullet points for why a user might consider using the app
🤖 > - Generate unique and personalized profile pictures - Choose from a variety of styles to suit your mood or personality - Stand out from the crowd with AI-generated images - Say goodbye to boring profile pictures
🤔 > Give me some options for text that goes on the call-to-action button on the landing page for this app
🤖 > - "Generate my profile picture" - "Try it now" - "Get started" - "Create my image" - "Make my profile picture" - "Pick my style"
Example 3: Writing prompts and inspiration⌗
See it on ShareGPTSounds like you won’t be staring at a blank page for much longer.
🤔 > I want a few short writing prompts about a sci-fi story in a post-apocalyptic setting
🤖 > 1. After a catastrophic event wipes out most of humanity, a small group of survivors must band together to navigate the dangerous new world and search for a way to rebuild society. 2. In a world where the air is toxic and resources are scarce, a young woman must journey across the ruins of a once-great city to find a mysterious device that could hold the key to their survival. 3. In the aftermath of a massive alien invasion, a small group of humans must fight to survive against the extraterrestrial threat and the treacherous landscape of a post-apocalyptic world. 4. In a future where technology has advanced beyond recognition, a group of rebels must use their unique abilities to overthrow an oppressive government and restore freedom to the people. 5. After a devastating war between rival factions, a lone survivor must navigate the treacherous landscape of a post-apocalyptic world and confront the demons of their past in order to find a way to start anew.
Example 4: Emails⌗
🤔 > Write an email to my English Literature professor asking for help on an issue I'm having with my thesis
🤖 > Dear Professor, I am writing to request your help with an issue I am having with my thesis. I am having difficulty organizing my ideas and developing a clear argument. I was wondering if you could offer some guidance or suggestions on how to improve my thesis. I would greatly appreciate any advice you have to offer. Thank you for your time and consideration. Sincerely, [Your Name]
AI Art⌗
Let’s see if we can get ChatGPT to generate prompts that we can directly use to generate AI Art using Midjourney.
🤔 > Describe an image that shows a futuristic utopian city in vivid detail under one paragraph
🤖 > In the image, the futuristic city is bustling with activity as flying cars zip through the air above gleaming skyscrapers. The buildings are sleek and modern, with intricate architectural designs and vibrant, neon-lit windows. The streets are clean and free of pollution, with trees and greenery lining the sidewalks. People of all ages and backgrounds can be seen walking, talking, and going about their business, giving the city a vibrant and diverse feel. In the distance, a massive space elevator can be seen reaching up into the sky, signaling the city's advanced technology and progress. Overall, the city exudes a sense of prosperity, progress, and harmony.
I then used that output on Midjourney v4:
🤔 > /imagine futuristic city is bustling with activity as flying cars zip through the air above gleaming skyscrapers. The buildings are sleek and modern, with intricate architectural designs and vibrant, neon-lit windows. The streets are clean and free of pollution, with trees and greenery lining the sidewalks. People of all ages and backgrounds can be seen walking, talking, and going about their business, giving the city a vibrant and diverse feel. In the distance, a massive space elevator can be seen reaching up into the sky, signaling the city's advanced technology and progress --v 4
And here are the stunning results (I ran it 4 times with the same prompt):
Recommendations⌗
Wouldn’t be great if you had a friend knowledgeable in many different fields that you could ask for recommendations on anything? Well now you do, sort of.
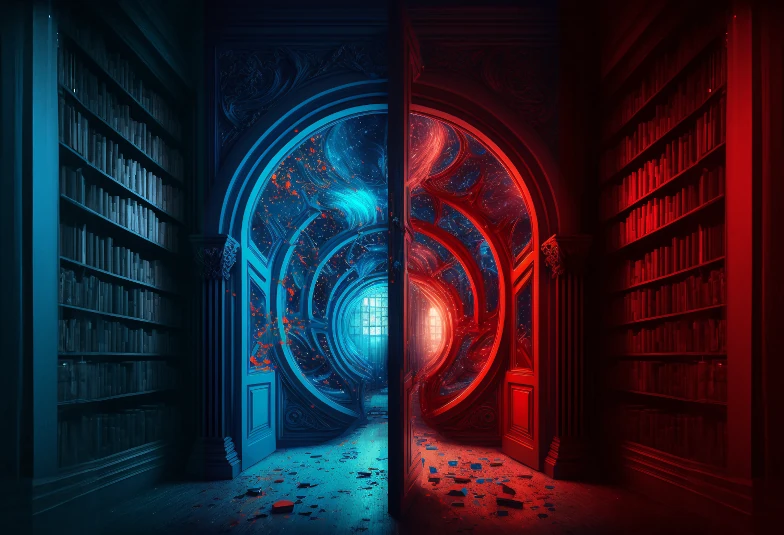
Jump to:
Example 1: Books⌗
🤔 > What are some books like "The Pragmatic Programmer" ?
🤖 > Here are some books that are similar to "The Pragmatic Programmer": - "Clean Code" by Robert C. Martin "Code Complete" by Steve McConnell "The Art - of Readable Code" by Dustin Boswell and Trevor Foucher "Refactoring: - Improving the Design of Existing Code" by Martin Fowler "The Clean Coder: A - Code of Conduct for Professional Programmers" by Robert C. Martin All of these books focus on best practices and techniques for writing high-quality, maintainable code. They cover a range of topics, including design, testing, debugging, and refactoring.
🤔 > Give more titles
🤖 > Here are some additional titles that might be of interest: - "The Mythical Man-Month" by Frederick P. Brooks Jr. - "Design Patterns: Elements of Reusable Object-Oriented Software" by Erich - Gamma, Richard Helm, Ralph Johnson, and John Vlissides - "Working Effectively with Legacy Code" by Michael Feathers - "Agile Software Development, Principles, Patterns, and Practices" by Robert C. Martin - "Don't Make Me Think: A Common Sense Approach to Web Usability" by Steve Krug - "How to Solve It: A New Aspect of Mathematical Method" by George Pólya - "The Logic of Computer Science" by Kees Doets and Jan van Eijck - "Structure and Interpretation of Computer Programs" by Harold Abelson and Gerald Jay Sussman - "Introduction to the Theory of Computation" by Michael Sipser These books cover a wide range of topics in computer science and software engineering. Some focus on specific areas, such as design patterns or usability, while others provide a broader overview of the field. All of them offer valuable insights and advice for software developers.
Example 2: Musicians⌗
🤔 > What are some artists that make music similar in to Ludovico Einaudi ?
🤖 > Ludovico Einaudi is a contemporary classical composer and pianist, known for his minimalist and evocative style. Some artists who make music similar to his include Max Richter, Olafur Arnalds, and Nils Frahm. All of these musicians are known for creating beautiful and emotive music that often incorporates elements of classical, ambient, and electronic music.
Example 3: YouTube Channels⌗
🤔 > Give me YouTube channels that do engineering projects, similar to "Stuff Made Here" and "Wintergatan"
🤖 > There are many YouTube channels that feature engineering projects and experiments. Some popular ones include "The King of Random," "Mark Rober," and "Adam Savage's Tested." These channels feature a wide variety of engineering projects, from simple DIY experiments to more complex builds and inventions. If you're looking for something similar to "Stuff Made Here" and "Wintergatan," I would recommend checking out these channels.
🤔 > Do you have more?
🤖 > Yes, there are many other YouTube channels that feature engineering projects and experiments. Some other popular ones include "Smarter Every Day," "The Backyard Scientist," and "NightHawkInLight." These channels offer a unique blend of science, engineering, and experimentation, and are sure to provide plenty of inspiration for your own projects. I would also recommend checking out "Practical Engineering," which features in-depth explanations of engineering principles and how they are applied in real-world projects. Additionally, "The Q" is a great channel for anyone interested in engineering and technology, as it features a wide variety of projects and experiments that showcase the latest advances in these fields.
Language Learning⌗
ChatGPT can also be a great tool for learning languages and enriching your vocabulary.
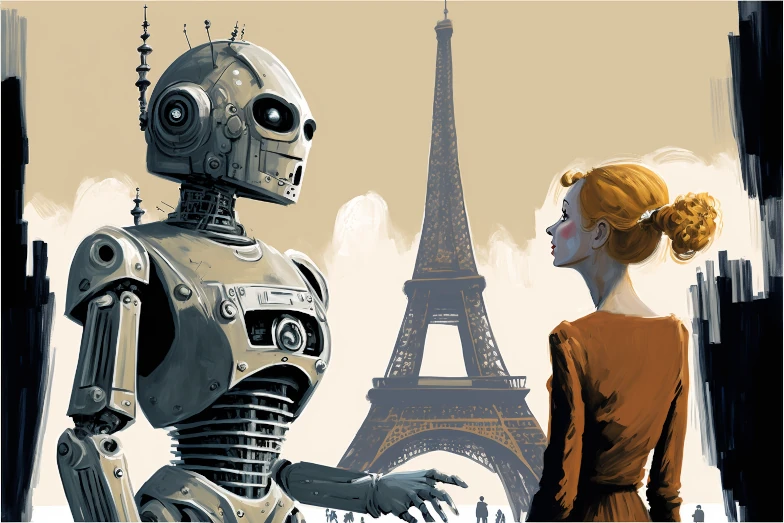
Take a look at this example (ShareGPT):
🤔 > I'm trying to learn French, I have very basic understanding of the language. I want to ask you questions in English and you reply in French using words a beginner would understand, also provide the English translation just in case I don't understand. Can you do that ?
🤖 > Oui, je peux vous aider à apprendre le français. Je vais répondre à vos questions en utilisant des mots simples que les débutants peuvent comprendre. Si vous ne comprenez pas quelque chose, n'hésitez pas à me demander une traduction en anglais. Voici quelques phrases de base en français pour vous aider à démarrer : - Bonjour ! Comment ça va ? (Hello! How are you?) - Je m'appelle... (My name is...) - Je viens des États-Unis. (I come from the United States.) - Je suis ici pour apprendre le français. (I am here to learn French.) - Je suis débutant en français. (I am a beginner in French.) - Pouvez-vous parler plus lentement, s'il vous plaît ? (Can you speak more slowly, please?) N'hésitez pas à me poser d'autres questions si vous avez besoin d'aide pour apprendre le français. Je suis là pour vous aider !
🤔 > How are you doing today?
🤖 > Je vais bien, merci. Comment allez-vous aujourd'hui ? (I'm doing well, thank you. How are you today?)
🤔 > Doing great, having fun learning a new language! What did you have for breakfast ?
🤖 > Je suis un programme informatique, je ne mange pas de petit déjeuner. Mais je suis toujours là pour vous aider à apprendre le français ! (I'm a computer program, I don't eat breakfast. But I'm always here to help you learn French!)
🤔 > Tell me a short joke
🤖 > Voici une blague courte en français : Pourquoi est-ce qu'un pneu est triste ? Parce qu'il est dégonflé ! (Here is a short joke in French: Why is a tire sad? Because it's deflated!) La traduction en anglais : Why is a tire sad? Because it's deflated!
🤔 > Thanks, I gotta go, talk to you soon!
🤖 > De rien, c'était un plaisir de vous aider à apprendre le français. Au revoir ! (You're welcome, it was a pleasure to help you learn French. Goodbye!)
Some Other Shenanigans⌗
ChatGPT is capable of so much more, and the examples shown here so far are
just scratching the surface of what it’s capable of.
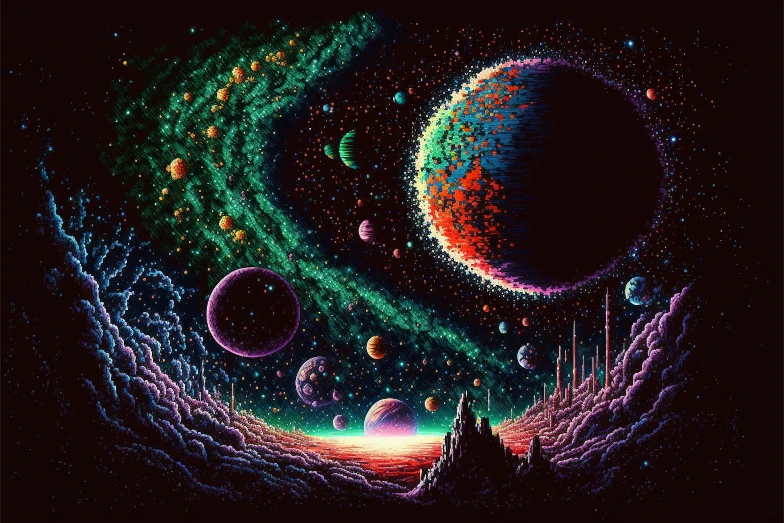
Jump to:
Example 1: Write songs⌗
🤔 > Write a 1940s country song about the hardships of being a programmer
🤖 > In a time before computers were widespread, When coding was a new and daunting task, We sat and stared at lines of code all day, Trying to make the machine do our bidding, But oh, the hardships we faced back then. We battled bugs and glitches all night long, Our fingers cramped from typing on the keys, Our eyes strained from staring at the screen, But still we pressed on, driven by our dreams, To make the machine bend to our will. But oh, the hardships we faced back then, With no Google to search for our answers, No Stack Overflow to guide our way, We had to figure it out on our own, And oh, the hardships we faced back then. But still we persevered, day after day, Until at last our code was running smooth, And though the hardships may have weighed us down, We never let them keep us from our goals, And oh, the pride we felt back then. So here's a toast to all the programmers, Who've faced the hardships and come out on top, We may have struggled, but we never gave up, And though the road was rough, we made it through, And oh, the hardships we faced back then.
Example 2: Explain like I’m 5⌗
🤔 > Explain to me like I'm 5 years old how robots are made
🤖 > Sure, I'd be happy to explain how robots are made! Here's a simple explanation: robots are made by people called engineers using a lot of different parts. The parts might include things like metal or plastic for the body, motors to make the robot move, sensors to help the robot see or hear, and a computer brain to help the robot make decisions. The engineers put all of these parts together to make a robot that can do different tasks. For example, a robot might be able to pick up and move objects, or it might be able to talk to people. It's kind of like building a toy, but with a lot more advanced parts!
Example 3: Summarize long text⌗
I asked ChatGPT to summarize one part of Vannevar Bush’s essay “As We May Think}”. See it on ShareGPT
🤔 > Please give me a summary of the following text: "Of what lasting benefit has been man's use of science and of the new instruments which his research brought into existence? First, they have increased his control of his material environment. They have improved his food, his clothing, his shelter; they have increased his security and released him partly from the bondage of bare existence. They have given him increased knowledge of his own biological processes so that he has had a progressive freedom from disease and an increased span of life. They are illuminating the interactions of his physiological and psychological functions, giving the promise of an improved mental health. Science has provided the swiftest communication between individuals; it has provided a record of ideas and has enabled man to manipulate and to make extracts from that record so that knowledge evolves and endures throughout the life of a race rather than that of an individual. There is a growing mountain of research. But there is increased evidence that we are being bogged down today as specialization extends. The investigator is staggered by the findings and conclusions of thousands of other workers—conclusions which he cannot find time to grasp, much less to remember, as they appear. Yet specialization becomes increasingly necessary for progress, and the effort to bridge between disciplines is correspondingly superficial."
🤖 > The use of science and new technology has improved human life by increasing control over the environment, improving health and longevity, facilitating communication, and providing a way to record and build upon knowledge. However, the increasing specialization of research is creating a barrier to further progress.
Seen in the Wild⌗
Examples I’ve seen on Twitter and elsewhere:
Slides⌗
- The ChatGPT Prompt Book: A set of interesting prompt examples from Dr Alan D. Thompson.
Videos⌗
- What if OpenAI did Blender scripts?: Generate Blender Python scripts.
Articles⌗
- ChatGPT and the future of software engineering: Using ChatGPT to define a new programming language and instruct it to write programs in that language. Also shows examples of just telling ChatGPT to come up with its own language.
- Disputing A Parking Fine with ChatGPT
- Jailbreaking ChatGPT on Release Day
- Building A Virtual Machine inside ChatGPT: Jonas Degrave shows how ChatGPT can simulate a linux shell and even create a virtual machine!
- Dart & Flutter with ChatGPT: Is it worth it?: Using ChatGPT to generate Dart & Flutter code.
Twitter⌗
- Twitter Thread of known ChatGPT jailbreaks: Some ways people have successfully bypassed ChatGPT’s filters.
- Examples showing Where ChatGPT fails
- Parse search result: Extract information from a search result
- #ChatGPT Twitter hashtag: You can find plenty of examples on Twitter.
Are you enjoying this post?
Get notified when I publish new posts:
Tips & Tricks⌗
Okay, now that you’ve seen plenty of examples that show the potential of ChatGPT, let’s have a look at some tips and tricks that’ll help you get the output you want.
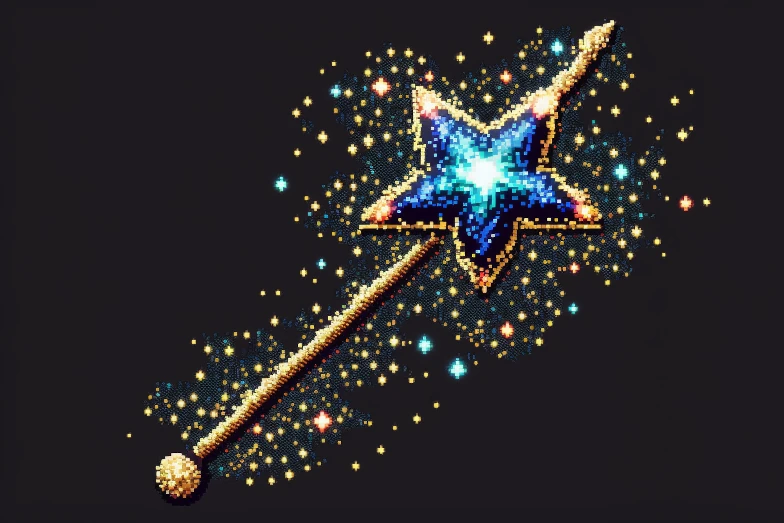
Output cutoff⌗
The output produced by ChatGPT sometimes cuts off when it’s too long, here you
can try saying: continue output
or continue where you left off
and it will
usually finish writing where it stopped.
Getting better answers⌗
If you don’t get the result you expected, you can either press the Try again button
or send a new prompt to guide it into giving you the result you want.
Get more details⌗
When ChatGPT returns a list and you want more detail on one or more items, you can always ask it to elaborate.
Example:
🤔 > Give me a list of short helpful tips to improve my writing style
🤖 > 1. Start by using simple, clear, and concise language. [...] 3. Use specific, concrete examples and evidence to support your points. This will help your writing be more persuasive and convincing. [...] 7. Don't be afraid to revise and edit your work. Writing is often a process of trial and error, and it's important to take the time to refine and improve your writing. [...] 10. Finally, remember that practice makes perfect. [...]
🤔 > Elaborate on 3 and 7
🤖 > 1. Using specific, concrete examples and evidence to support your points is an important part of effective writing. This helps to make your writing more persuasive and convincing, as it shows that you have thought carefully about your argument and [...]. 2. Revising and editing your work is an important part of the writing process. Writing is often a process of trial and error, and it's important to take the time to [...].
Limitations⌗
While the examples I’ve shown here are very impressive, it is important to keep in mind that ChatGPT does have some limitations:
- It can produce answers that sound plausible but are in fact incorrect, misleading or nonsensical. 1
- It might fail at seemingly simple logical questions, since it currently doesn’t have access to any source of truth. 2
- Answers are not always consistent. You can prompt it for something and it claims it doesn’t know the answer, but if you try rephrasing your prompt you might get a correct answer.
- It can be verbose and repetitive, for example it will often remind you that it’s a large language model trained by OpenAI:
🤖 > I am a large language model trained by OpenAI, so I have a lot of general knowledge, but [...]
- While OpenAI has filters in place that limits the model’s output, you might still be able to get it to respond to “inappropriate requests” with the right prompt, or it might produce insensitive, unsafe or harmful content.
- You don’t necessarily get the same answers if you use the same prompts.
Tools / Resources⌗
Communities⌗
- /r/chatGPT: ChatGPT’s subreddit.
Prompts / Repositories⌗
- LearnGPT: Lists examples of prompts and their output
- awesome-chatgpt-prompts: Github repo containing curated list of prompts
- humanloop/awesome-chatgpt: Links to helpful ChatGPT resources.
Browser extensions⌗
- ShareGPT: Browser extension to share ChatGPT threads (instead of having to rely on screenshots).
- Display ChatGPT results alongside Google results: Browser extension to show ChatGPT output alongside Google search result pages.
Bots⌗
Scripts / Wrappers⌗
- acheong08/ChatGPT: Python wrapper for the ChatGPT API
Impact & Reception⌗
The response from ChatGPT’s release varies from complete bewilderment to skepticism or outright calling for OpenAI to shut down the public access 3.
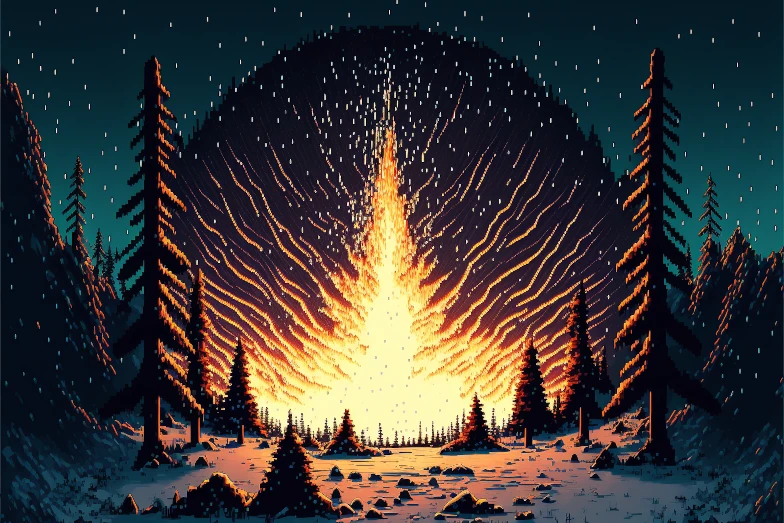
Response⌗
- StackOverflow introduces a new policy banning the influx of ChatGPT-generated answers because of concerns about their quality and accuracy. (Explanation)
- HackerNews discussion on banning ChatGPT-generated content
Press⌗
- Wired: ChatGPT, Galactica, and the Progress Trap
- The Verge: ChatGPT proves AI is finally mainstream — and things are only going to get weirder
- Mashable: The ChatGPT chatbot from OpenAI is amazing, creative, and totally wrong
- TechCrunch: Is ChatGPT a ‘virus that has been released into the wild’?
Videos⌗
- Two Minute Papers: OpenAI ChatGPT: The Future Is Here!
- ColdFusion: It’s Time to Pay Attention to A.I. (ChatGPT and Beyond): Excellent video covering the impact ChatGPT has had in the last few weeks and what can we expect in the future.
- Yannic Kilcher: ChatGPT: This AI has a JAILBREAK?! (Unbelievable AI Progress)
- MKBHD: The Truth About AI Getting “Creative”
Conclusion⌗
I think we’ve seen enough in this post to say AI has come a long way and is
proving to be very useful in various domains.
Will this (and
subsequent iterations of this technology) change the way we work forever? I
don’t know, I think the long-term effects of this technology will become
apparent as more time goes by.
I would love to hear what you think of ChatGPT? Do you think this would be useful for your work or other areas of your life? Let me know in the comments below!
Changelog⌗
My aim for this post is to be a definitive resource for ChatGPT and have a list of tools and resources that relate to it. I’ll try to keep it up to date as much as I can. If you think something should be in this post, please let me know (either in the comments here or on Twitter) and I’ll try to add it in.
In this section I’ll state the changes made to the post and the date they were made.
12/22/22
⌗
- Updated Seen in the Wild and Impact & Reception sections.
12/13/22
⌗
- Updated Learn More and Seen in the Wild sections.
12/12/22
⌗
- Updated Learn More, Impact & Reception and Tools / Resources sections.
12/11/22
⌗
- Initial publication
Get notified when I publish new posts: